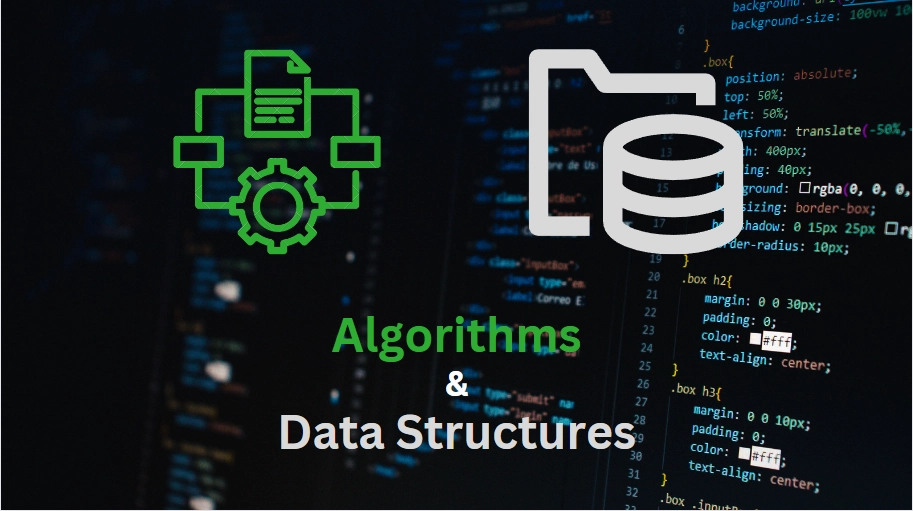
Mastering Algorithms and Data Structures for Beginners: A Step-by-Step Guide and Complete Roadmap
Ouhadj ilyes
Software Engineer
Web Development
Category
Feb. 22, 2023
Updated on
This article is meant for absolute beginners that struggle to find the exact steps and resources to start learning Algorithms & Data Structures. Hopefully, at the end of this article, you'll be able to make a curriculum for yourself to get a really good understanding of Algorithms & Data Structures that will eventually make you a much better programmer.
The resources I provide in this article have helped me and many other software engineers to be better at problem solving, writing better software designs as well as thoroughly thinking of efficiency and performance when writing code.
I will just assume you know at least the definition of both Algorithms and Data Structures.
Without further intros, let's get started !
Article Links
- Algorithms & Data Structures
Why Bother With Algorithms & Data Structures ?
Algorithms and data structures are essential for all of the different roles and specialties in computer science and here's 3 reasons why you need to consider at least mastering the basics:
-
Improve Problem Solving Capabilities
An algorithm in its core definition is just the exact steps of solving a particular problem, and coding problems involve analyzing, storing and manipulating data, that's where comes the need for data structures which are nothing but “meaningful” arrangements of that data. However, sometimes there's different solutions for the same problem, algorithms will not only teach you how to solve problems but also how to find the best solutions for your problems.
-
Build Efficinet & Optimized Software
As I just mentioned, when there's different solutions for the same coding problem, the main goal of algorithms and data structures is to find the best solution that takes less time as well as less space in memory, and that's what efficient and performant software is all about.
-
Ace Job Interviews
Organizations such as Google and Facebook look for employees who have a good grasp of data structures and algorithms, and questions about such skills are frequently asked in job interviews.
CS50 : Start Here !
CS50 is an on-campus and online introductory course on computer science taught at Harvard University and Yale University. If you are an absolute beginner, the first thing to consider is an introduction to the world of algorithms and data structures, but while you find all the introductory resources online pretty boring and just making things more complicated, CS50 have got the best 2 introductory videos on this planet on algorithms and Data Structures.
Their way of explaining things is pretty simple and brilliant that anyone new to this field will feel comfortable learning these two skills. This has to be the best place to start from to get a really good grasp of the foundations.
You can watch the videos on their official website here. you can also find them on this Youtube Playlist on their channel.
Grokking Algorithms
Even though you can start from here as well, but I will highly recommend watching the CS50 videos on algorithms and data structures, they will give you a great understanding of the foundations of those two skills, and grasping the intermediate or advanced topics would be much easier.
After grasping the foundations, the next step would be reading a book about algorithms and data structures, not a detailed textbook or a 1000-pages boring book, but Grokking Algorithms is the friendliest and easiest book to read ever written on algorithms and data structures, it's meant for absolute beginners so if you exposed yourself to the advanced topics so far then you are not going to learn much from this book.
Grokking Algorithms is a fully illustrated, friendly guide that teaches you how to apply algorithms and data structures to the practical problems you face every day as a programmer. it stands out with its really simple explanations and lots of real life examples and illustrations, it uses python-based code samples and over 400 pictures with detailed walkthroughs. But remember to practice, and make sure to complete all the exercises at the end of each chapter before looking at their solutions.
Once you finish reading this book, you'll be comfortable enough to study the advanced topics on your own (check out the Roadmap below), you may also consider reading a detailed textbook if you are into reading books. I will recommend reading Introduction to Algorithms by Thomas H. Cormen
Roadmap : Do It On Your Own
Once you consume the resources I provided in the previous sections or if you are not a beginner and already acquire the core concepts and foundations, here's a detailed roadmap for mastering algorithms and data structures, to help you find out where you are at your current stage and where to go next. I will also provide some links to resources that may be helpful to you to learn each topic on your own.
-
Prerequisites : Phase 0
The only prerequisite before learning algorithms and data structures is having some prior knowledge of at least one programming language, if you have no prior experience with any programming languages, I will recommend starting with Python as it is one of the most popular and easiest languages to learn and pick up quickly.
If you choose to go with python, you will only need to grasp the basics at least, here's a list of some of the python basics that you will most likely need before starting to learn algorithms and data structures:
- Variables
- Conditions
- Chained Conditionals
- Operators
- Control Flow (If/Else)
- Loops and Iterables
- Functions
- Mutable vs. Immutable
- Common Methods
- File IO
- ...
Resources for Python basics:
- Docs : Python Official Docs
- Book : Python Crash Course
- Online Course : A Beginners Guide to Python Programming
If you are not comfortable reading books or documentations, you can find great content on Youtube to learn the basics of Python.
-
Core Algorithm Concepts : Phase 1
-
Big-O Analysis
- O-notation. Ω-notation. θ-notation
- Asymptotic Notation
- Standard Notations
- Common Functions
- O-notation. Ω-notation. θ-notation
-
Algorithm Design
- Reasoning About Correctness
- Modeling The Problem
- Proof By Contradiction
- Estimation
-
Sorting
- Elementary Sorts
- Mergesort
- Quicksort
- Priority Queues
-
Searching
- Symbol Tables
- Binary Search
- Balanced Search
- Hash Tables
-
Recursion
- Base Case & Recursive Case
- The Stack
- Top-Down Recursion
- The Staircase Problem
-
Divide & Conquer
- Multiplying Square Matrices
- Strassen's algorithm
- Solving Recurrences
- Proof of the continuous master theorem
-
-
Intermediate Topics : Phase 2
-
Two Pointers
- Two Pointer Technique
- Analysis
- Implementation
-
Binary Trees
- Binary Search Trees
- Analyzing Performance of Binary search Trees
- Using Binary Tree as (Key, Value) Symbol Table
- Using The Binary Tree as a Priority Queue
-
Backtracking
- Backtracking Technique
- Backtracking Algorithms
-
Dynamic Programming
- Rod Cutting
- Matrix-chain multiplication
- Elements of dynamic programming
- Longest common subsequence
- Optimal binary search trees
-
Greedy Algorithms
- Activity-selection problems
- Elements of the greedy strategy
- Huffman codes
- Offline caching
-
Amortized Analysis
- Aggregate analysis
- The accounting method
- The potential method
- Dynamic tables
-
-
Advanced Concepts : Phase 3
-
Graph Algorithms
- Elementary Graph Algorithms
- Minimum Spanning Trees
- Single-Source Shortest Paths
- All-Pairs Shortest Paths
- Maximum Flow
- Matchings in Bipartite Graphs
-
Parallel Algorithms
- The basics of fork-join parallelism
- Parallel matrix multiplication
- Parallel merge sort
-
Online Algorithms
- Waiting for an elevator
- Maintaining a search list
- Online caching
-
Matrix Operations
- Solving systems of linear equations
- Inverting matrices
- Symmetric positive-definite matrices and least-squares approximation
-
Linear Programming
- Linear programming formulations and algorithms
- Formulating problems as linear programs
- Duality
-
Polynomials and the FFT
- Representing polynomials
- The DFT and FFT
- FFT circuits
-
Number-Theoretic Algorithms
- Elementary number-theoretic notions
- Greatest common divisor
- Modular arithmetic
- The RSA public-key cryptosystem
- Primality testing
-
String Matching
- The naive string-matching algorithm
- The Rabin-Karp algorithm
- The Knuth-Morris-Pratt algorithm
- Suffix arrays
-
Approximation Algorithms
- The vertex-cover problem
- The traveling-salesperson problem
- The set-covering problem
- Randomization and linear programming
- The subset-sum problem
-
-
Data Structures : Phase 4
Basic & Intermediate Data Structures:
-
Elementary Data Structures
- Arrays, matrices, stacks, queues
- Linked Lists
- Rooted Trees
-
Hash Tables
- Direct-address tables
- Hash tables
- Hash functions
- Open addressing
-
Red-Black Trees
- Properties of red-black trees
- Rotations
- Insertion
- Deletion
Advanced Data Structures:
-
Augmenting Data Structures
- Dynamic order statistics
- How to augment a data structure
- Interval trees
-
B-Trees
- What is a B-Tree
- Operations on B-Trees
- Deleting a key from a B-Tree
-
Data Structures for Disjoint Sets
- Disjoint-set operations
- Linked-list representations of disjoint sets
- Disjoint-set forests
- Analysis of union by rank with path compression
-
-
Roadmap Learning Resources:
-
Books
Each one of these books covers from one to many topics listed on the Roadmap, by reading all of them you'll have covered all of the topics of this Roadmap (Make sure to practice for as much as possible, you can finish all of them in 1-2 weeks but without practicing, you are just wasting your time):
-
Online Courses
Some people prefer watching and listening rather than reading, but a combination of those two will make learning and grasping the concepts deeper and much easier. Here's some online courses that can help:
-
Conclusion
Now we would like to hear your thoughts, if you need more help or have any questions, feel free to reach out to us using the Contact Form or by Email and we would be happy to help !